KSampler
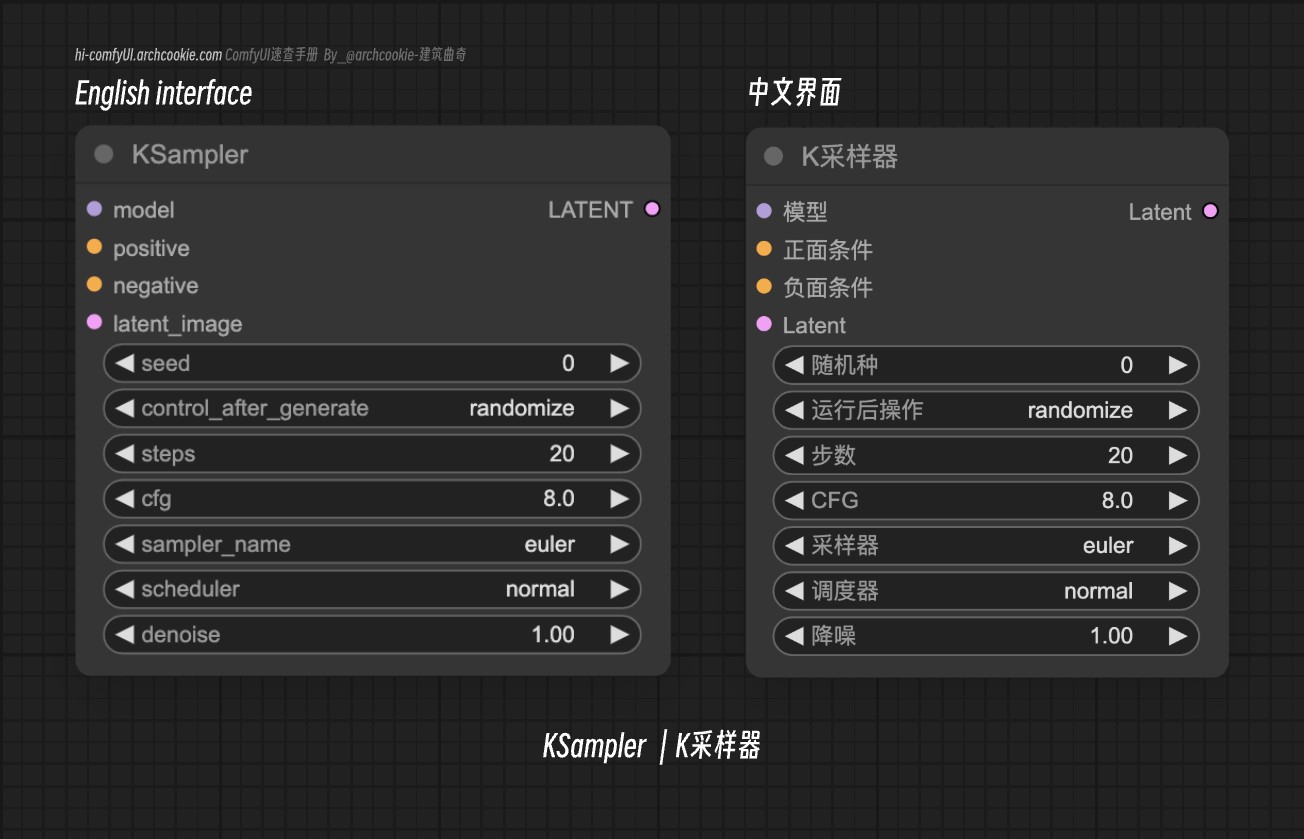
Documentation
- Class name:
KSampler
- Category:
sampling
- Output node:
False
The KSampler node is designed for advanced sampling operations within generative models, allowing for the customization of sampling processes through various parameters. It facilitates the generation of new data samples by manipulating latent space representations, leveraging conditioning, and adjusting noise levels.
Input types
Parameter | Comfy dtype | Description |
---|---|---|
model | MODEL | Specifies the generative model to be used for sampling, playing a crucial role in determining the characteristics of the generated samples. |
seed | INT | Controls the randomness of the sampling process, ensuring reproducibility of results when set to a specific value. |
steps | INT | Determines the number of steps to be taken in the sampling process, affecting the detail and quality of the generated samples. |
cfg | FLOAT | Adjusts the conditioning factor, influencing the direction and strength of the conditioning applied during sampling. |
sampler_name | COMBO[STRING] | Selects the specific sampling algorithm to be used, impacting the behavior and outcome of the sampling process. |
scheduler | COMBO[STRING] | Chooses the scheduling algorithm for controlling the sampling process, affecting the progression and dynamics of sampling. |
positive | CONDITIONING | Defines positive conditioning to guide the sampling towards desired attributes or features. |
negative | CONDITIONING | Specifies negative conditioning to steer the sampling away from certain attributes or features. |
latent_image | LATENT | Provides a latent space representation to be used as a starting point or reference for the sampling process. |
denoise | FLOAT | Controls the level of denoising applied to the samples, affecting the clarity and sharpness of the generated images. |
Output types
Parameter | Comfy dtype | Description |
---|---|---|
latent | LATENT | Represents the latent space output of the sampling process, encapsulating the generated samples. |